Créer une Calculatrice 3D Simple avec HTML, CSS et JavaScript
Code Source Gratuit
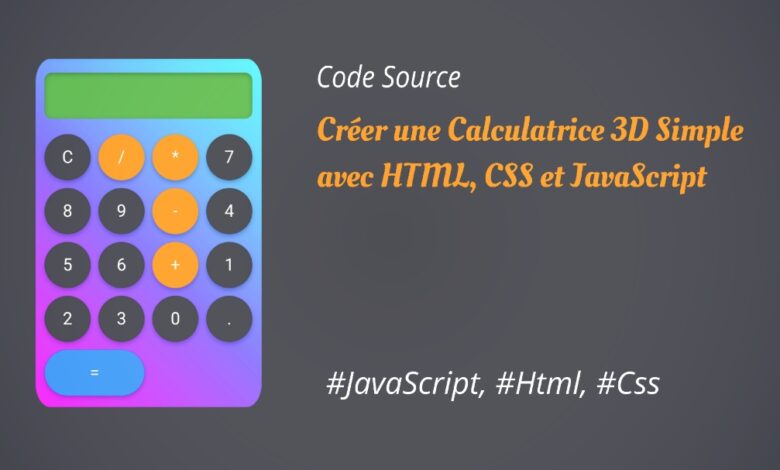
💻 Nouveau sur le blog : Code source d’une calculatrice 3D en HTML, CSS et JavaScript ! Idéale pour s’initier à la programmation 3D, cette calculatrice est conçue pour être simple et facile à personnaliser.
Explorez comment manipuler des éléments en trois dimensions et découvrez les bases des transformations CSS et des calculs JavaScript.
Code Source
<!DOCTYPE html>
<html lang="fr">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Calculatrice 3D Animée</title>
<style>
body {
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #1a1a1a;
font-family: Arial, sans-serif;
overflow: hidden;
}
.calculator {
background-color: #333;
border-radius: 20px;
padding: 20px;
box-shadow:
0 0 20px rgba(0,0,0,0.3),
inset 0 0 10px rgba(255,255,255,0.1),
0 5px 15px rgba(0,0,0,0.5);
transition: transform 0.3s ease;
position: relative;
z-index: 10;
}
.calculator::before {
content: '';
position: absolute;
top: -5px;
left: -5px;
right: -5px;
bottom: -5px;
background: linear-gradient(45deg, #ff00ff, #00ffff);
z-index: -1;
border-radius: 25px;
filter: blur(5px);
}
.calculator:hover {
transform: scale(1.05) rotateY(10deg);
}
#display {
width: 100%;
height: 60px;
margin-bottom: 20px;
background-color: #4CAF50;
border: none;
border-radius: 10px;
font-size: 24px;
text-align: right;
padding: 10px;
box-sizing: border-box;
color: white;
transition: background-color 0.3s ease;
box-shadow: inset 0 2px 5px rgba(0,0,0,0.2);
}
.buttons {
display: grid;
grid-template-columns: repeat(4, 1fr);
gap: 10px;
}
button {
width: 60px;
height: 60px;
font-size: 20px;
border: none;
border-radius: 50%;
background-color: #555;
color: white;
cursor: pointer;
transition: all 0.3s ease;
box-shadow: 0 3px 5px rgba(0,0,0,0.2);
}
button:hover {
background-color: #777;
transform: scale(1.1) translateY(-3px);
box-shadow: 0 5px 10px rgba(0,0,0,0.3);
}
button:active {
transform: scale(0.95) translateY(1px);
box-shadow: 0 2px 3px rgba(0,0,0,0.2);
}
.operator {
background-color: #FF9800;
}
.operator:hover {
background-color: #FFA726;
}
#equals {
background-color: #2196F3;
grid-column: span 2;
width: 100%;
border-radius: 30px;
}
#equals:hover {
background-color: #42A5F5;
}
.ball {
position: absolute;
border-radius: 50%;
opacity: 0.7;
animation: floatBall 15s infinite linear;
}
</style>
</head>
<body>
<div class="calculator">
<input type="text" id="display" readonly>
<div class="buttons">
<button onclick="clearDisplay()">C</button>
<button onclick="appendToDisplay('/')" class="operator">/</button>
<button onclick="appendToDisplay('*')" class="operator">*</button>
<button onclick="appendToDisplay('7')">7</button>
<button onclick="appendToDisplay('8')">8</button>
<button onclick="appendToDisplay('9')">9</button>
<button onclick="appendToDisplay('-')" class="operator">-</button>
<button onclick="appendToDisplay('4')">4</button>
<button onclick="appendToDisplay('5')">5</button>
<button onclick="appendToDisplay('6')">6</button>
<button onclick="appendToDisplay('+')" class="operator">+</button>
<button onclick="appendToDisplay('1')">1</button>
<button onclick="appendToDisplay('2')">2</button>
<button onclick="appendToDisplay('3')">3</button>
<button onclick="appendToDisplay('0')">0</button>
<button onclick="appendToDisplay('.')">.</button>
<button onclick="calculate()" id="equals">=</button>
</div>
</div>
<script>
function appendToDisplay(value) {
document.getElementById('display').value += value;
}
function clearDisplay() {
document.getElementById('display').value = '';
}
function calculate() {
try {
const result = eval(document.getElementById('display').value);
document.getElementById('display').value = result;
animateResult();
} catch (error) {
document.getElementById('display').value = 'Erreur';
}
}
function animateResult() {
const display = document.getElementById('display');
display.style.backgroundColor = '#2196F3';
setTimeout(() => {
display.style.backgroundColor = '#4CAF50';
}, 300);
}
// Animation des boutons au chargement
window.onload = function() {
const buttons = document.querySelectorAll('button');
buttons.forEach((button, index) => {
button.style.opacity = '0';
button.style.transform = 'translateY(20px)';
setTimeout(() => {
button.style.transition = 'all 0.5s ease';
button.style.opacity = '1';
button.style.transform = 'translateY(0)';
}, index * 50);
});
// Création des boules animées
createBalls();
}
function createBalls() {
const colors = ['#ff00ff', '#00ffff', '#ffff00', '#ff0000', '#00ff00', '#0000ff'];
for (let i = 0; i < 20; i++) {
const ball = document.createElement('div');
ball.classList.add('ball');
ball.style.width = `${Math.random() * 30 + 10}px`;
ball.style.height = ball.style.width;
ball.style.backgroundColor = colors[Math.floor(Math.random() * colors.length)];
ball.style.left = `${Math.random() * 100}vw`;
ball.style.top = `${Math.random() * 100}vh`;
ball.style.animationDuration = `${Math.random() * 10 + 5}s`;
ball.style.animationDelay = `${Math.random() * 5}s`;
document.body.appendChild(ball);
}
}
</script>
<style>
@keyframes floatBall {
0% {
transform: translateY(100vh) scale(0);
}
100% {
transform: translateY(-100px) scale(1);
}
}
</style>
</body>
</html>
Télécharger le Code Source
🔢 Découvrez une calculatrice 3D simple, conçue en HTML, CSS et JavaScript !
Ce code source est parfait pour ceux qui veulent s’initier à la programmation 3D ou créer leurs propres projets interactifs.
Avec une structure claire et facile à personnaliser, ce projet vous permettra de manipuler des éléments en 3D et de mieux comprendre le fonctionnement des transformations CSS et des calculs JavaScript.
Téléchargez, expérimentez et développez vos compétences !
Téléchargez le code et laissez libre cours à votre créativité !